Creative Coding Week 2: Exercise 1 - Vera Molnar's 25 Squares
An example of Vera Molnar's art was supplied in the course material. Our goal was to write code to generate something similar allowing us to apply our own interpretation of the piece. Ver Molnar's orinal piece follows with my 'interpretations' lower down
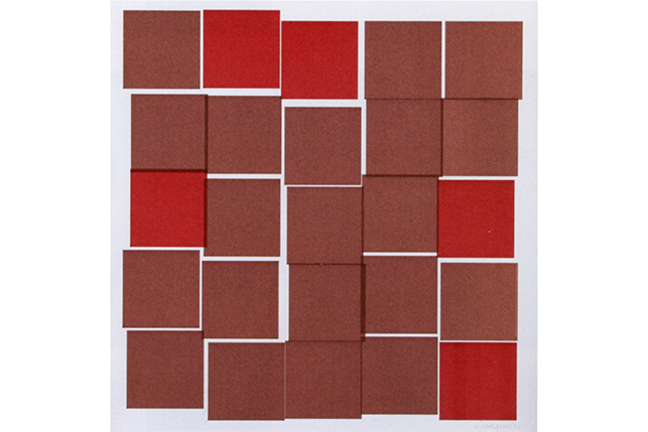
© Vera Molnar 1991
My first sketch recreates the piece with little interpretation.
> View source code for preceding sketch.
/*
* Creative Coding
* Week 2, Molnar 25 squares - first attempt
* by Gerard Holden
*
* Based on Week 2, 03 - n squares
* by Indae Hwang and Jon McCormack
*
* The program displays 25 squares in a grid, each square
* is offset by a small random amount. The squares are either brown
* (0.8 probability) or red (0.2 probability). The program repeats
* with each iteration a different arrangement.
*/
float border; // Distance from squares to window border
float sqlen; // Dimensions of squares
float gap = 10; //The initial gap between cells
float randLo = -10; //The low end of the jiggle range
float randHi = 10; // The high end of the jiggle range
int randCol; //Random number used to determin colour of square
float x, y; //Coordinates of square
void setup(){
size(600,600);
rectMode(CORNER);
noStroke();
frameRate(0.5);
border = width/16; // Distance from squares to window border
sqlen = (width - border * 2)/5; // Dimensions of squares
randomSeed(hour()+minute()+second()); //Randomise the seed
}
void draw() {
background(192);
for (int i = 4; i >= 0; i--) { // Draw in reverse so shadows not covered
for (int j = 4; j >= 0; j--) {
x = border + sqlen * i + random(randLo, randHi);
y = border + sqlen * j + random(randLo, randHi);
//Draw shadow
fill(128,100);
rect(x + 5, y + 5, sqlen-gap, sqlen-gap);
//Select colour of square
randCol = int(random(5)); //Pick a number between 0 and 4
if (randCol == 0) { //If number is zero, square is red else brown
fill(179, 32, 32);
} else {
fill(140, 68, 65);
}
//Display square
rect(x, y, sqlen-gap, sqlen-gap);
}
}
// save your drawing when you press keyboard 's'
if (keyPressed == true && key == 's') {
saveFrame("25Squares.jpg");
}
}
My second sketch contains some animation between each set of squares. Through this exercise I gained an appreciation of frameRate, smooth animation and to use pauses.
> View source code for preceding sketch.
/*
* Creative Coding
* Week 2, Molnar 25 squares - second attempt
* by Gerard Holden
*
* Based on Week 2, 03 - n squares
* by Indae Hwang and Jon McCormack
*
* The program displays 25 squares in a grid, each square
* is offset by a small random amount. The squares are either brown
* (0.8 probability) or red (0.2 probability). The program repeats
* with a different arrangement for each iteration. The program incliudes
* an animation sequence when drawing the squares.
*
*/
float[][] msquare = new float[25][5]; // An array to store square data
float border; // Distance from squares to window border
float cellwidth; // Dimension of cell in which square is placed
float sqwidth; // Dimension of the square
float sqlen; // Dimensions of squares
float gap = 10; //The initial gap between cells
float randLo = -10; //The low end of the jiggle range
float randHi = 10; // The high end of the jiggle range
int randCol; //Random number used to determin colour of square
float x, y; //Coordinates of square
long lastTime; // Used to include a pause at end of each cycle
int XHOME = 0;
int YHOME = 1;
int XOFF = 2;
int YOFF = 3;
int SQCOL = 4;
int step = -1;
color colour1, colour2, colourbkgd; // Colours for squares and background
float xstart, ystart; // Starting position of animation
void setup(){
size(600,600);
rectMode(CORNER);
noStroke();
colorMode(RGB, 255);
// Set colours
colour1 = color(140, 68, 65); // Brown
colour2 = color(179, 32, 32); // Red
colourbkgd = color(192, 192, 192); //Gray
// Calculate some dimensions
border = min(width,height)/16; // Distance from squares to window border
cellwidth = (min(width,height) - border * 2)/5; // Dimensions of squares
sqwidth = cellwidth - gap;
randomSeed(hour()+minute()+second()); //Randomise the seed
// Set start point for animation
xstart = width/4;
ystart = height/4;
// Calculate position of each square (before random jiggle set)
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
msquare[i*5 + j][XHOME] = border + cellwidth*i;
msquare[i*5 + j][YHOME] = border + cellwidth*j;
msquare[i*5 + j][SQCOL] = (int) random(5);
}
}
// A variable used to pause animation
lastTime = millis();
// Set start point for animation
xstart = width/2 - cellwidth/2;
ystart = height/2 - cellwidth/2;
smooth();
}
void draw() {
background(colourbkgd);
// A step counter is used to allow squares to animate
step += 1;
if (step > 100) {
step = -1;
}
// Randomise jiggle and color at start of each cycle
if (step == 0) {
for (int i = 24; i >= 0; i--) {
msquare[i][XOFF] = msquare[i][XHOME] + random(randLo, randHi);
msquare[i][YOFF] = msquare[i][YHOME] + random(randLo, randHi);
msquare[i][SQCOL] = (int) random(5);
}
}
// Draw squares
for (int i = 24; i >= 0; i--) {
x = xstart + (msquare[i][XOFF] - xstart) * step/100;
y = ystart + (msquare[i][YOFF] - ystart) * step/100;
fill(96,100);
rect(x + 5, y + 5, sqwidth, sqwidth);
fill(colour1);
if (msquare[i][SQCOL] == 0) {
fill(colour2);
}
rect(x, y, sqwidth, sqwidth);
}
// Pause after drawing squares at start point
if (step == 0) {
while (millis() - lastTime < 1000) {
}
lastTime = millis();
}
// Pause after drawing squares at their final destination
if (step == 100) {
while (millis() - lastTime < 5000) {
}
lastTime = millis();
}
}